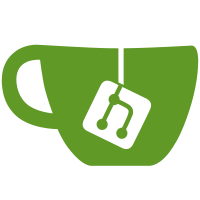
# Conflicts: # admin/admin.php # classes/Battles/User.php # classes/Battles/UserStats.php # fbattle.php # functions.php
803 lines
49 KiB
PHP
803 lines
49 KiB
PHP
<?php
|
||
|
||
use Battles\Chat;
|
||
use Battles\DressedItems;
|
||
use Battles\GameLogs;
|
||
use Battles\Nick;
|
||
use Battles\Template;
|
||
use Battles\User;
|
||
|
||
require_once "functions.php";
|
||
$user = User::getInstance();
|
||
$check = new class{
|
||
|
||
public static function getInTower(): bool
|
||
{
|
||
return \Battles\Database\Db::getInstance()->fetchColumn('select in_tower from users where id = ?', User::getInstance()->getId()) === 1;
|
||
}
|
||
};
|
||
|
||
if (!$check::getInTower()) {
|
||
header('Location: main.php');
|
||
exit;
|
||
}
|
||
$rooms[0] = '';
|
||
|
||
$rhar = [
|
||
"501" => [20, 0, 502, 505, 0],
|
||
"502" => [15, 0, 0, 0, 501],
|
||
"503" => [15, 0, 0, 507, 0],
|
||
"504" => [15, 0, 0, 508, 0],
|
||
"505" => [20, 501, 0, 510, 0],
|
||
"506" => [15, 0, 507, 511, 0],
|
||
"507" => [15, 503, 508, 0, 506],
|
||
"508" => [25, 504, 0, 513, 507],
|
||
"509" => [20, 0, 0, 515, 0],
|
||
"510" => [20, 505, 511, 0, 0],
|
||
"511" => [20, 506, 0, 0, 510],
|
||
"512" => [30, 0, 513, 519, 0],
|
||
"513" => [25, 508, 514, 0, 512],
|
||
"514" => [20, 0, 0, 0, 513],
|
||
"515" => [20, 509, 0, 522, 0],
|
||
"516" => [25, 0, 517, 523, 0],
|
||
"517" => [25, 0, 518, 0, 516],
|
||
"518" => [35, 0, 519, 525, 517],
|
||
"519" => [35, 512, 520, 526, 518],
|
||
"520" => [35, 0, 521, 0, 519],
|
||
"521" => [15, 0, 0, 528, 0],
|
||
"522" => [20, 515, 0, 529, 0],
|
||
"523" => [15, 516, 0, 530, 0],
|
||
"524" => [20, 0, 525, 531, 0],
|
||
"525" => [35, 518, 526, 532, 524],
|
||
"526" => [40, 519, 527, 533, 525],
|
||
"527" => [35, 0, 0, 0, 526],
|
||
"528" => [15, 521, 529, 535, 0],
|
||
"529" => [20, 522, 0, 0, 528],
|
||
"530" => [20, 523, 531, 537, 0],
|
||
"531" => [35, 524, 0, 538, 530],
|
||
"532" => [20, 525, 533, 539, 0],
|
||
"533" => [20, 526, 534, 540, 532],
|
||
"534" => [15, 0, 0, 0, 533],
|
||
"535" => [20, 528, 0, 541, 0],
|
||
"536" => [20, 0, 537, 0, 535],
|
||
"537" => [35, 530, 0, 543, 536],
|
||
"538" => [20, 531, 0, 544, 0],
|
||
"539" => [20, 532, 0, 545, 0],
|
||
"540" => [15, 533, 0, 546, 0],
|
||
"541" => [20, 535, 542, 547, 0],
|
||
"542" => [15, 0, 543, 0, 541],
|
||
"543" => [40, 537, 0, 549, 542],
|
||
"544" => [40, 538, 545, 550, 543],
|
||
"545" => [40, 539, 0, 551, 544],
|
||
"546" => [15, 540, 0, 552, 0],
|
||
"547" => [20, 541, 548, 553, 0],
|
||
"548" => [20, 0, 549, 0, 547],
|
||
"549" => [35, 543, 550, 0, 548],
|
||
"550" => [40, 544, 551, 554, 549],
|
||
"551" => [40, 545, 0, 555, 550],
|
||
"552" => [15, 546, 0, 556, 0],
|
||
"553" => [20, 547, 0, 557, 0],
|
||
"554" => [20, 550, 555, 0, 0],
|
||
"555" => [35, 551, 0, 0, 554],
|
||
"556" => [15, 552, 0, 559, 0],
|
||
"557" => [15, 553, 0, 0, 0],
|
||
"558" => [20, 0, 559, 0, 0],
|
||
"559" => [20, 556, 560, 0, 558],
|
||
"560" => [20, 0, 0, 0, 559],
|
||
];
|
||
|
||
function get_out($u)
|
||
{
|
||
$pers = db::c()->query('SELECT * FROM users WHERE id = ?i', $u)->fetch_assoc_array();
|
||
DressedItems::undressAllItems($pers['id']);
|
||
db::c()->query('UPDATE inventory SET owner = ?i WHERE owner = ?i', $pers['id'], $pers['id'] + _BOTSEPARATOR_);
|
||
###
|
||
$row = db::c()->query('SELECT * FROM `effects` WHERE `owner` = ?i', $pers['id'] + _BOTSEPARATOR_);
|
||
$travm = [11, 12, 13, 14];
|
||
while ($efs = $row->fetch_assoc()) {
|
||
if (in_array($efs['type'], $travm)) {
|
||
$pers['sila'] -= $efs['sila'];
|
||
$pers['lovk'] -= $efs['lovk'];
|
||
$pers['inta'] -= $efs['inta'];
|
||
$pers['vinos'] -= $efs['vinos'];
|
||
} else {
|
||
$pers['sila'] += $efs['sila'];
|
||
$pers['lovk'] += $efs['lovk'];
|
||
$pers['inta'] += $efs['inta'];
|
||
$pers['vinos'] += $efs['vinos'];
|
||
$pers['maxhp'] += $efs['hp'];
|
||
}
|
||
$efs['owner'] = $_SESSION['uid'];
|
||
//mysql_query('UPDATE `effects` SET `owner` = "' . $efs['owner'] . '" WHERE `id` = "' . $efs['id'] . '" LIMIT 1');
|
||
//mysql_query('UPDATE `users` SET `sila` = "' . $pers['sila'] . '", `lovk` = "' . $pers['lovk'] . '", `inta` = "' . $pers['inta'] . '", `vinos` = "' . $pers['vinos'] . '", `maxhp` = "' . $pers['maxhp'] . '" WHERE `id` = "' . $pers['id'] . '" LIMIT 1');
|
||
}
|
||
###
|
||
}
|
||
|
||
mysql_query("LOCK TABLES `bots` WRITE, `deztow_stavka` WRITE, `users` WRITE, `deztow_items` WRITE, `inventory` WRITE, `battle` WRITE, `logs` WRITE, `deztow_turnir` WRITE, `effects` WRITE,`shop` WRITE, `online` WRITE, `deztow_gamers_inv` WRITE, `deztow_realchars` WRITE, `deztow_eff` WRITE, `variables` WRITE");
|
||
|
||
$ls = mysql_num_rows(mysql_query("SELECT `id` FROM `users` WHERE `bot` = 1 and `in_tower` = 1"));
|
||
$kol_pl = mysql_num_rows(mysql_query("SELECT `id` FROM `users` WHERE `bot` = 0 and `in_tower` = 1"));
|
||
$tur_data = mysql_fetch_array(mysql_query("SELECT * FROM `deztow_turnir` WHERE `active` = TRUE"));
|
||
|
||
if ($_GET['give']) {
|
||
$obj = mysql_fetch_array(mysql_query("SELECT * FROM `deztow_items` WHERE `id` = '" . mysql_real_escape_string($_GET['give']) . "' and `room` = '" . $user->getRoom() . "' LIMIT 1"));
|
||
if ($obj) {
|
||
if ($_SESSION['timei'] - time() <= 0) {
|
||
$_SESSION['timei'] = (time() + 3);
|
||
$dress = mysql_fetch_array(mysql_query("SELECT * FROM `shop` WHERE `id` = '" . $obj['iteam_id'] . "' LIMIT 1"));
|
||
if (isset($dress['id'])) {
|
||
mysql_query("INSERT INTO `inventory` (`bs`, `prototype`, `owner`, `name`, `type`, `massa`, `cost`, `img`, `maxdur`, `isrep`, `gsila`, `glovk`, `ginta`, `gintel`, `ghp`, `gnoj`, `gtopor`, `gdubina`, `gmech`, `gfire`, `gwater`, `gair`, `gearth`, `glight`, `ggray`, `gdark`, `needident`, `nsila`, `nlovk`, `ninta`, `nintel`, `nmudra`, `nvinos`, `nnoj`, `ntopor`, `ndubina`, `nmech`, `nfire`, `nwater`, `nair`, `nearth`, `nlight`, `ngray`, `ndark`, `mfkrit`, `mfakrit`, `mfuvorot`, `mfauvorot`, `bron1`, `bron2`, `bron3`, `bron4`, `maxu`, `minu`, `magic`, `nlevel`, `nalign`, `dategoden`, `goden`, `otdel`, `koll`) VALUES ('1', '{$dress['id']}', '{$user['id']}', '{$dress['name']}', '{$dress['type']}', '{$dress['massa']}', '{$dress['cost']}', '{$dress['img']}', '{$dress['maxdur']}', '{$dress['isrep']}', '{$dress['gsila']}', '{$dress['glovk']}', '{$dress['ginta']}', '{$dress['gintel']}', '{$dress['ghp']}', '{$dress['gnoj']}', '{$dress['gtopor']}', '{$dress['gdubina']}', '{$dress['gmech']}', '{$dress['gfire']}', '{$dress['gwater']}', '{$dress['gair']}', '{$dress['gearth']}', '{$dress['glight']}', '{$dress['ggray']}', '{$dress['gdark']}', '{$dress['needident']}', '{$dress['nsila']}', '{$dress['nlovk']}', '{$dress['ninta']}', '{$dress['nintel']}', '{$dress['nmudra']}', '{$dress['nvinos']}', '{$dress['nnoj']}', '{$dress['ntopor']}', '{$dress['ndubina']}', '{$dress['nmech']}', '{$dress['nfire']}', '{$dress['nwater']}', '{$dress['nair']}', '{$dress['nearth']}', '{$dress['nlight']}', '{$dress['ngray']}', '{$dress['ndark']}', '{$dress['mfkrit']}', '{$dress['mfakrit']}', '{$dress['mfuvorot']}', '{$dress['mfauvorot']}', '{$dress['bron1']}', '{$dress['bron3']}', '{$dress['bron2']}', '{$dress['bron4']}', '{$dress['maxu']}', '{$dress['minu']}', '{$dress['magic']}', '{$dress['nlevel']}', '{$dress['nalign']}', '" . (($dress['goden']) ? ($dress['goden'] * 24 * 60 * 60 + time()) : "") . "', '{$dress['goden']}', '{$dress['razdel']}', '{$dress['koll']}')");
|
||
mysql_query("DELETE FROM `deztow_items` WHERE `id` = '" . mysql_real_escape_string($_GET['give']) . "' and `room` = '" . $user->getRoom() . "' LIMIT 1");
|
||
} else {
|
||
echo '<font color=red>Предмет не найден. Сообщите Администрации данный код #' . $obj['iteam_id'] . '#</font>';
|
||
}
|
||
} else {
|
||
echo "<font color=red>Вы сможете поднять вещь через " . ($_SESSION['timei'] - time()) . " секунд...</font>";
|
||
}
|
||
} else {
|
||
echo "<font color=red>Кто-то был быстрее...</font>";
|
||
}
|
||
}
|
||
|
||
if ($_POST['attack']) {
|
||
$jert = mysql_fetch_array(mysql_query("SELECT `id`, `login`, `room`, `battle`, `hp` FROM `users` WHERE `login` = '" . mysql_real_escape_string($_POST['attack']) . "' LIMIT 1"));
|
||
if ($jert['room'] == $user->getRoom() && $jert['id'] != $user['id']) {
|
||
if ($jert['id'] == 233 || $jert['id'] == 234 || $jert['id'] == 235) {
|
||
$arha = mysql_fetch_array(mysql_query('SELECT * FROM `bots` WHERE `prototype` = "' . $jert['id'] . '" LIMIT 1'));
|
||
if (isset($arha['id'])) {
|
||
$jert['battle'] = $arha['battle'];
|
||
$jert['id'] = $arha['id'];
|
||
} else {
|
||
$jert['battle'] = 0;
|
||
#$jert['id'] = $jert['id']; ?!?!?!?!?!?!
|
||
}
|
||
$bot = 1;
|
||
}
|
||
|
||
if ($jert['battle'] > 0) {
|
||
$bd = mysql_fetch_array(mysql_query('SELECT * FROM `battle` WHERE `id` = "' . $jert['battle'] . '" LIMIT 1'));
|
||
$battle = unserialize($bd['teams']);
|
||
$ak = array_keys($battle[$jert['id']]);
|
||
$battle[$user['id']] = $battle[$ak[0]];
|
||
foreach ($battle[$user['id']] as $k => $v) {
|
||
$battle[$k][$user['id']] = [0, 0, time()];
|
||
$battle[$user['id']][$k] = [0, 0, time()];
|
||
}
|
||
$t1 = explode(";", $bd['t1']);
|
||
if (in_array($jert['id'], $t1)) {
|
||
$ttt = 2;
|
||
$ttt2 = 1;
|
||
} else {
|
||
$ttt = 1;
|
||
$ttt2 = 2;
|
||
}
|
||
addch("<b>" . Nick::id($user['id'])->short() . "</b> вмешался в <a href=logs.php?log=" . $id . " target=_blank>поединок »»</a>.", $user->getRoom());
|
||
GameLogs::addBattleLog($jert['battle'], '<span class=date>' . date("H:i") . '</span> ' . Nick::id($user['id'])->short() . ' вмешался в поединок!<BR>');
|
||
mysql_query('UPDATE `battle` SET `teams` = \'' . serialize($battle) . '\', `t' . $ttt . '` = CONCAT(`t' . $ttt . '`,\';' . $user['id'] . '\'), `to' . $ttt . '` = \'' . time() . '\', `to' . $ttt2 . '` = \'' . (time() - 1) . '\' WHERE `id` = "' . $jert['battle'] . '" LIMIT 1');
|
||
mysql_query("UPDATE `users` SET `battle` = '" . $jert['battle'] . "', `zayavka` = 0 WHERE `id` = '" . $user['id'] . "' LIMIT 1");
|
||
mysql_query('UPDATE `deztow_turnir` SET `log` = CONCAT(`log`,\'' . "<span class=date>" . date("d.m.y H:i") . "</span> " . Nick::id($user['id'])->full(1) . " вмешался в поединок против " . Nick::id($jert['id'])->full(1) . " <a href=\"logs.php?log={$jert['battle']}\" target=_blank>»»</a><BR>" . '\') WHERE `active` = TRUE LIMIT 1');
|
||
header("Location: fbattle.php");
|
||
} else {
|
||
if ($bot) {
|
||
mysql_query("INSERT INTO `bots` (`name`, `prototype`, `battle`, `hp`) values ('" . $jert['login'] . "', '" . $jert['id'] . "', '', '" . $jert['hp'] . "')");
|
||
$jert['id'] = mysql_insert_id();
|
||
}
|
||
|
||
$teams = [];
|
||
$teams[$user['id']][$jert['id']] = [0, 0, time()];
|
||
$teams[$jert['id']][$user['id']] = [0, 0, time()];
|
||
$sv = [1, 2, 3, 4, 5];
|
||
mysql_query("INSERT INTO `battle` (`coment`, `teams`, `timeout`, `type`, `status`, `t1`, `t2`, `to1`, `to2`, `blood`) VALUES ('', '" . serialize($teams) . "', '" . $sv[rand(0, 3)] . "', '10', '0', '" . $user['id'] . "', '" . $jert['id'] . "', '" . time() . "', '" . time() . "', '1')");
|
||
$id = mysql_insert_id();
|
||
|
||
if ($bot) {
|
||
mysql_query("UPDATE `bots` SET `battle` = {$id} WHERE `id` = {$jert['id']} LIMIT 1");
|
||
} else {
|
||
mysql_query("UPDATE `users` SET `battle` = {$id} WHERE `id` = {$jert['id']} LIMIT 1");
|
||
}
|
||
|
||
$rr = "<b>" . Nick::id($user['id'])->full(1) . "</b> и <b>" . Nick::id($jert['id'])->full(1) . "</b>";
|
||
addch("<B><b>" . Nick::id($user['id'])->short() . "</b> , применив магию нападения, внезапно напал на <b>" . Nick::id($jert['id'])->short() . "</b>.", $user->getRoom());
|
||
GameLogs::addBattleLog($id, "Часы показывали <span class=date>" . date("Y.m.d H.i") . "</span>, когда " . $rr . " бросили вызов друг другу. <BR>");
|
||
mysql_query("UPDATE `users` SET `battle` = {$id}, `zayavka` = 0 WHERE (`id` = {$user['id']} OR `id` = {$jert['id']})");
|
||
mysql_query('UPDATE `deztow_turnir` SET `log` = CONCAT(`log`,\'' . "<span class=date>" . date("d.m.y H:i") . "</span> " . Nick::id($user['id'])->full(1) . " напал на " . Nick::id($jert['id'])->full(1) . " завязался <a href=\"logs.php?log={$id}\" target=_blank>бой »»</a><BR>" . '\') WHERE `active` = TRUE LIMIT 1');
|
||
header("Location: fbattle.php");
|
||
}
|
||
} else {
|
||
echo '<font color=red>Жертва ускользнула из комнаты...</font>';
|
||
}
|
||
}
|
||
|
||
$_GET['path'] = (int)$_GET['path'];
|
||
if ($rhar[$user->getRoom()][$_GET['path']] > 0 && $_GET['path'] < 5 && $_GET['path'] > 0 && ($_SESSION['time'] <= time())) {
|
||
$rr = mysql_fetch_array(mysql_query("SELECT * FROM `effects` WHERE `type` = 10 AND `owner` = {$user['id']} LIMIT 1"));
|
||
if (!isset($rr['id'])) {
|
||
$list = mysql_query("SELECT `id`, `room`, `login` FROM `users` WHERE `room` = '" . $user->getRoom() . "' AND `in_tower` = 1");
|
||
while ($u = mysql_fetch_array($list)) {
|
||
if ($u['id'] != $user['id']) {
|
||
addchp('<font color=red>Внимание!</font> <b>' . $user['login'] . '</b> отправился в <b>' . $rooms[$rhar[$user->getRoom()][$_GET['path']]] . '</b>.', '{[]}' . $u['login'] . '{[]}');
|
||
}
|
||
}
|
||
|
||
$list = mysql_query("SELECT `id`, `room`, `login` FROM `users` WHERE `room` = '" . $rhar[$user->getRoom()][$_GET['path']] . "' AND `in_tower` = 1");
|
||
while ($u = mysql_fetch_array($list)) {
|
||
addchp('<font color=red>Внимание!</font> <B>' . $user['login'] . '</B> вошел в комнату.', '{[]}' . $u['login'] . '{[]}');
|
||
}
|
||
mysql_query("UPDATE `users`, `online` SET `users`.`room` = '" . $rhar[$user->getRoom()][$_GET['path']] . "', `online`.`room` = '" . $rhar[$user->getRoom()][$_GET['path']] . "' WHERE `online`.`id` = `users`.`id` AND `online`.`id` = '{$user['id']}'");
|
||
$_SESSION['time'] = (time() + $rhar[$rhar[$user->getRoom()][$_GET['path']]][0]);
|
||
header('Location: towerin.php');
|
||
} else {
|
||
err('Вы парализованы и не можете двигаться...');
|
||
}
|
||
}
|
||
|
||
$list = mysql_query("SELECT * FROM `users` WHERE `in_tower` = 1 AND `battle` = 0");
|
||
while ($u = mysql_fetch_array($list)) {
|
||
if ($u['hp'] <= 0) {
|
||
DressedItems::undressAllItems($u['id']);
|
||
$rep = mysql_query("SELECT * FROM `inventory` WHERE `owner` = '" . $u['id'] . "' AND `bs` = 1");
|
||
while ($r = mysql_fetch_array($rep)) {
|
||
mysql_query("INSERT `deztow_items` (`iteam_id`, `name`, `img`, `room`) VALUES ('" . $r['prototype'] . "', '" . $r['name'] . "', '" . $r['img'] . "', '" . $u['room'] . "')");
|
||
}
|
||
|
||
mysql_query("DELETE FROM `inventory` WHERE `owner` = '" . $u['id'] . "' AND `bs` = 1");
|
||
|
||
$tec = mysql_fetch_array(mysql_query("SELECT * FROM `deztow_realchars` WHERE `owner` = '{$u['id']}' LIMIT 1"));
|
||
if (isset($tec['id'])) {
|
||
mysql_query("DELETE FROM `deztow_realchars` WHERE `owner` = '{$u['id']}'");
|
||
$u = mysql_fetch_array(mysql_query("SELECT * FROM `users` WHERE `id` = '{$u['id']}' LIMIT 1"));
|
||
mysql_query("UPDATE `users` SET `sila` = '" . $tec['sila'] . "', `lovk` = '" . $tec['lovk'] . "', `inta` = '" . $tec['inta'] . "', `vinos` = '" . $tec['vinos'] . "', `intel` = '" . $tec['intel'] . "', `stats` = '" . $tec['stats'] . "', `nextup` = '" . $tec['nextup'] . "', `level` = '" . $tec['level'] . "', `hp` = '" . ($tec['vinos'] * 6) . "', `maxhp` = '" . ($tec['vinos'] * 6) . "', `align` = '" . $tec['align'] . "', `noj` = '" . $tec['noj'] . "', `mec` = '" . $tec['mec'] . "', `topor` = '" . $tec['topor'] . "', `dubina` = '" . $tec['dubina'] . "', `mlight` = '" . $tec['mlight'] . "', `mgray` = '" . $tec['mgray'] . "', `mdark` = '" . $tec['mdark'] . "', `master` = '" . $tec['master'] . "' WHERE `id` = '" . $u['id'] . "' LIMIT 1");
|
||
}
|
||
|
||
$eff = mysql_fetch_array(mysql_query("SELECT * FROM `effects` WHERE `owner` = '" . $u['id'] . "' AND (`type` = 11 OR `type` = 12 OR `type` = 13 OR `type` = 14 OR `type` = 2 OR `type` = 3)"));
|
||
mysql_query("DELETE FROM `effects` WHERE `owner` = '" . $u['id'] . "' AND `type` != 11 AND `type` != 12 AND `type` != 13 AND `type` != 14 AND `type` != 2 AND `type` != 3");
|
||
|
||
if ($tec[0]) {
|
||
mysql_query("UPDATE `users` SET `sila` = `sila`-'" . $eff['sila'] . "', `lovk` = `lovk`-'" . $eff['lovk'] . "', `inta` = `inta`-'" . $eff['inta'] . "' WHERE `id` = '" . $eff['owner'] . "' LIMIT 1");
|
||
}
|
||
get_out($u['id']);
|
||
|
||
mysql_query("UPDATE `users` SET `in_tower` = 0, `room` = '31' WHERE `id` = '" . $u['id'] . "'");
|
||
mysql_query("UPDATE `online` SET `room` = '31' WHERE `id` = '" . $u['id'] . "'");
|
||
|
||
mysql_query('UPDATE `deztow_turnir` SET `log` = CONCAT(`log`,\'' . "<span class=date>" . date("d.m.y H:i") . "</span> " . Nick::id($u['id'])->full(1) . " повержен и выбывает из турнира<br />" . '\') WHERE `active` = TRUE LIMIT 1');
|
||
addchp('<font color=red>Внимание!</font> Вы выбыли из турнира Башни Смерти.', '{[]}' . $u['login'] . '{[]}');
|
||
header('Location: tower.php');
|
||
}
|
||
}
|
||
|
||
if (($kol_pl + $ls) < 2 && ($tur_data['start_time'] + 60) <= time()) {
|
||
$tur = mysql_fetch_array(mysql_query("SELECT * FROM `deztow_turnir` WHERE `active` = TRUE LIMIT 1"));
|
||
DressedItems::undressAllItems($user['id']);
|
||
$rep = mysql_query("SELECT * FROM `inventory` WHERE `owner` = '" . $user['id'] . "' AND `bs` = 1");
|
||
while ($r = mysql_fetch_array($rep)) {
|
||
mysql_query("INSERT `deztow_items` (`iteam_id`, `name`, `img`, `room`) VALUES ('" . $r['prototype'] . "', '" . $r['name'] . "', '" . $r['img'] . "', '" . $user->getRoom() . "');");
|
||
}
|
||
mysql_query("DELETE FROM `inventory` WHERE `owner` = '" . $user['id'] . "' AND `bs` = 1");
|
||
$tec = mysql_fetch_array(mysql_query("SELECT * FROM `deztow_realchars` WHERE `owner` = '{$user['id']}'"));
|
||
if (isset($tec['id'])) {
|
||
mysql_query("DELETE FROM `deztow_realchars` WHERE `owner` = '{$user['id']}'");
|
||
$u = mysql_fetch_array(mysql_query("SELECT * FROM `users` WHERE `id` = '{$user['id']}' LIMIT 1"));
|
||
mysql_query("UPDATE `users` SET `sila` = '" . $tec['sila'] . "', `lovk` = '" . $tec['lovk'] . "', `inta` = '" . $tec['inta'] . "', `vinos` = '" . $tec['vinos'] . "', `intel` = '" . $tec['intel'] . "', `stats` = '" . $tec['stats'] . "', `nextup` = '" . $tec['nextup'] . "', `level` = '" . $tec['level'] . "', `align` = '" . $tec['align'] . "', `hp` = '" . ($tec['vinos'] * 6) . "', `maxhp` = '" . ($tec['vinos'] * 6) . "', `noj` = '" . $tec['noj'] . "', `mec` = '" . $tec['mec'] . "', `topor` = '" . $tec['topor'] . "', `dubina` = '" . $tec['dubina'] . "', `mlight` = '" . $tec['mlight'] . "', `mgray` = '" . $tec['mgray'] . "', `mdark` = '" . $tec['mdark'] . "', `master` = '" . $tec['master'] . "' WHERE `id` = '" . $user['id'] . "' LIMIT 1");
|
||
}
|
||
|
||
mysql_query("DELETE FROM `effects` WHERE `owner` = '" . $user['id'] . "' AND `type` != 11 AND `type` != 12 AND `type` != 13 AND `type` != 14 AND `type` != 2 AND `type` != 3");
|
||
get_out($user['id']);
|
||
mysql_query("UPDATE `users` SET `money` = (`money`+'" . $tur['coin'] . "'), `in_tower` = 0, `room` = 31 WHERE `id` = '" . $user['id'] . "' LIMIT 1");
|
||
mysql_query("UPDATE `online` SET `room` = 31 WHERE `id` = '" . $user['id'] . "' LIMIT 1");
|
||
|
||
mysql_query('UPDATE `deztow_turnir` SET `winner` = \'' . $user['id'] . '\', `winnerlog` = \'' . Nick::id($user['id'])->full(1) . '\', `endtime` = \'' . time() . '\', `active` = FALSE, `log` = CONCAT(`log`,\'' . "<span class=date>" . date("d.m.y H:i") . "</span> Турнир завершен. Победитель : " . Nick::id($user['id'])->full(1) . " Приз : <b>" . $tur['coin'] . "</b> кр. <br />" . '\') WHERE `active` = TRUE LIMIT 1');
|
||
addchp('<font color=red>Внимание!</font> Поздравляем! Вы победитель турнира Башни смерти! Получаете <b>' . $tur['coin'] . '</b> кр.', '{[]}' . $user['login'] . '{[]}');
|
||
Chat::sendSys('Внимание! Битва в Башне Смерти завершена. Победитель:' . $user['login'] . ' [' . $user['level'] . ']');
|
||
if ($tur['art'] == 1) {
|
||
$bs_art = 0;
|
||
} else {
|
||
$bs_art = 0;
|
||
} #1
|
||
mysql_query('UPDATE `variables` SET `value` = "' . (time() + 60 * 60 * 1) . '", `bs_art` = "' . $bs_art . '" WHERE `var` = "startbs" LIMIT 1');
|
||
|
||
mysql_query("TRUNCATE TABLE `deztow_stavka`");
|
||
mysql_query("TRUNCATE TABLE `deztow_gamers_inv`");
|
||
mysql_query("TRUNCATE TABLE `deztow_items`");
|
||
mysql_query("TRUNCATE TABLE `deztow_trap`");
|
||
|
||
header('Location: tower.php');
|
||
}
|
||
|
||
mysql_query("UNLOCK TABLES");
|
||
if ($user['hp'] <= 0) {
|
||
header('Location: tower.php');
|
||
exit;
|
||
}
|
||
Template::header('towerin');
|
||
?>
|
||
<script>
|
||
let Hint3Name = '';
|
||
|
||
function findlogin(title, script, name) {
|
||
document.all("hint3").innerHTML = '<table width=100% cellspacing=1 cellpadding=0 bgcolor=CCC3AA><tr><td align=center><B>' + title + '</td><td width=20 align=right valign=top style="cursor: pointer" onclick="closehint3();"><BIG><B>x</td></tr><tr><td colspan=2>' +
|
||
'<form action="' + script + '" method=POST><table width=100% cellspacing=0 cellpadding=2 bgcolor=FFF6DD><tr><INPUT TYPE=hidden name=sd4 value="<? echo @$user['id']; ?>"><td colspan=2>' +
|
||
'Укажите логин персонажа:<small><BR>(можно щелкнуть по логину в чате)</TD></TR><TR><TD width=50% align=right><INPUT TYPE=text NAME="' + name + '"></TD><TD width=50%><INPUT TYPE="submit" value=" »» "></TD></TR></TABLE></FORM></td></tr></table>';
|
||
document.all("hint3").style.visibility = "visible";
|
||
document.all("hint3").style.left = 100;
|
||
document.all("hint3").style.top = 100;
|
||
document.all(name).focus();
|
||
Hint3Name = name;
|
||
}
|
||
|
||
function returned2(s) {
|
||
if (top.oldlocation != '') {
|
||
top.frames['main'].navigate(top.oldlocation + '?' + s + 'tmp=' + Math.random());
|
||
top.oldlocation = '';
|
||
} else {
|
||
top.frames['main'].navigate('main.php?' + s + 'tmp=' + Math.random());
|
||
}
|
||
}
|
||
|
||
function closehint3() {
|
||
document.all("hint3").style.visibility = "hidden";
|
||
Hint3Name = '';
|
||
}
|
||
</script>
|
||
<div id=hint4 class=ahint></div>
|
||
<TABLE width=100% cellspacing=0 cellpadding=0>
|
||
|
||
<TR>
|
||
<TD><?= Nick::id($user)->battle() ?></TD>
|
||
<TD class='H3' align=right><?= $rooms[$user->getRoom()]; ?>
|
||
<IMG SRC=i/tower/attack.gif WIDTH=66 HEIGHT=24 ALT="Напасть на..." style="cursor:hand"
|
||
onclick="findlogin('Напасть на','towerin.php','attack')">
|
||
</TD>
|
||
<TR>
|
||
<TD valign=top>
|
||
<FONT COLOR=red></FONT>
|
||
|
||
<?
|
||
|
||
$its = mysql_query("SELECT * FROM `deztow_items` WHERE `room` = '" . $user->getRoom() . "';");
|
||
if (mysql_num_rows($its) > 0) {
|
||
echo '<H4>В комнате разбросаны вещи:</H4>';
|
||
}
|
||
while ($it = mysql_fetch_array($its)) {
|
||
echo ' <A HREF="towerin.php?give=', $it['id'], '"><IMG SRC="i/sh/', $it['img'], '" ALT="Подобрать предмет \'', $it['name'], '\'"></A>';
|
||
}
|
||
|
||
?>
|
||
</TD>
|
||
<TD colspan=3 valign=top align=right nowrap>
|
||
<link href="css/towerin.css" rel="stylesheet" type="text/css">
|
||
<script type="text/javascript">
|
||
function fastshow2(content) {
|
||
const el = document.getElementById("mmoves");
|
||
const o = window.event.srcElement;
|
||
|
||
if (content == '') {
|
||
el.innerHTML = '';
|
||
}
|
||
if (content != '' && el.style.visibility != "visible") {
|
||
el.innerHTML = '<small>' + content + '</small>';
|
||
}
|
||
|
||
let x = window.event.clientX + document.documentElement.scrollLeft + document.body.scrollLeft - el.offsetWidth + 5;
|
||
let y = window.event.clientY + document.documentElement.scrollTop + document.body.scrollTop + 20;
|
||
|
||
if (x + el.offsetWidth + 3 > document.body.clientWidth + document.body.scrollLeft) {
|
||
x = (document.body.clientWidth + document.body.scrollLeft - el.offsetWidth - 5);
|
||
if (x < 0) {
|
||
x = 0
|
||
}
|
||
|
||
}
|
||
|
||
if (y + el.offsetHeight + 3 > document.body.clientHeight + document.body.scrollTop) {
|
||
y = (document.body.clientHeight + document.body.scrollTop - el.offsetHeight - 3);
|
||
if (y < 0) {
|
||
y = 0
|
||
}
|
||
|
||
}
|
||
|
||
if (x < 0) {
|
||
x = 0;
|
||
}
|
||
if (y < 0) {
|
||
y = 0;
|
||
}
|
||
el.style.left = x + "px";
|
||
el.style.top = y + "px";
|
||
if (el.style.visibility != "visible") {
|
||
el.style.visibility = "visible";
|
||
}
|
||
}
|
||
|
||
function hideshow() {
|
||
document.getElementById("mmoves").style.visibility = 'hidden';
|
||
}
|
||
|
||
let solo_store;
|
||
|
||
function solo(n, name) {
|
||
if (check_access() == true) {
|
||
window.location.href = '?path=' + n + '&rnd=' + Math.random();
|
||
} else if (name && n) {
|
||
solo_store = n;
|
||
const add_text = (document.getElementById('add_text') || document.createElement('div'));
|
||
add_text.id = 'add_text';
|
||
add_text.innerHTML = 'Вы перейдете в: <strong>' + name + '</strong> (<a href="#" onclick="return clear_solo();">отмена</a>)';
|
||
document.getElementById('ione').parentNode.parentNode.nextSibling.firstChild.appendChild(add_text);
|
||
ch_counter_color('red');
|
||
}
|
||
return false;
|
||
}
|
||
|
||
function clear_solo() {
|
||
document.getElementById('add_text').removeNode(true);
|
||
solo_store = false;
|
||
ch_counter_color('#00CC00');
|
||
return false;
|
||
}
|
||
|
||
let from_map = false;
|
||
|
||
function imover(im) {
|
||
im.filters.Glow.Enabled = true;
|
||
if (from_map == false && im.id.match(/mo_(\d)/) && document.getElementById('b' + im.id)) {
|
||
from_map = true;
|
||
document.getElementById('b' + im.id).runtimeStyle.color = '#666666';
|
||
from_map = false;
|
||
}
|
||
}
|
||
|
||
function imout(im) {
|
||
im.filters.Glow.Enabled = false;
|
||
if (from_map == false && im.id.match(/mo_(\d)/) && document.getElementById('b' + im.id)) {
|
||
from_map = true;
|
||
document.getElementById('b' + im.id).runtimeStyle.color = document.getElementById('b' + im.id).style.color;
|
||
from_map = false;
|
||
}
|
||
}
|
||
|
||
function bimover(im) {
|
||
if (from_map == false && document.getElementById(im.id.substr(1)) {
|
||
from_map = true;
|
||
imover(document.getElementById(im.id.substr(1)));
|
||
from_map = false;
|
||
}
|
||
}
|
||
|
||
function bimout(im) {
|
||
if (from_map == false && document.getElementById(im.id.substr(1))) {
|
||
from_map = true;
|
||
imout(document.getElementById(im.id.substr(1)));
|
||
from_map = false;
|
||
}
|
||
}
|
||
|
||
function bsolo(im) {
|
||
if (document.getElementById(im.id.substr(1))) {
|
||
document.getElementById(im.id.substr(1)).click();
|
||
}
|
||
return false;
|
||
}
|
||
|
||
function Down() {
|
||
top.CtrlPress = window.event.ctrlKey;
|
||
}
|
||
|
||
document.onmousedown = Down;
|
||
</script>
|
||
<style type="text/css">
|
||
img.aFilter {
|
||
filter: Glow(color=, Strength=, Enabled=0);
|
||
cursor: pointer;
|
||
}
|
||
|
||
hr {
|
||
height: 1px;
|
||
}
|
||
</style>
|
||
|
||
<table border="0" cellpadding="0" cellspacing="0">
|
||
<tr align="right" valign="top">
|
||
<td>
|
||
<table cellpadding="0" cellspacing="0" border="0" width="1">
|
||
<tr>
|
||
<td>
|
||
<div style="position:relative; cursor: pointer;" id="ione"><img
|
||
src="i/tower/<?= (500 + $user->getRoom()) ?>.jpg" alt="" border="1"/>
|
||
</div>
|
||
</td>
|
||
</tr>
|
||
<tr>
|
||
<td align="right">
|
||
<div align="right" id="btransfers">
|
||
<table cellpadding="0" cellspacing="0" border="0" id="bmoveto">
|
||
<tr>
|
||
<td bgcolor="#D3D3D3">
|
||
|
||
</td>
|
||
</tr>
|
||
</table>
|
||
</div>
|
||
</td>
|
||
</tr>
|
||
</table>
|
||
</td>
|
||
<td>
|
||
<table width="80" border="0" cellspacing="0" cellpadding="0">
|
||
<tr>
|
||
<td>
|
||
<table width="80" border="0" cellspacing="0" cellpadding="0">
|
||
<tr>
|
||
<td colspan="3" align="center"><img src="i/move/navigatin_46.gif"
|
||
width="80"
|
||
height="4"/></td>
|
||
</tr>
|
||
<tr>
|
||
<td colspan="3" align="center">
|
||
<table width="80" border="0" cellspacing="0" cellpadding="0">
|
||
<tr>
|
||
<td><img src="i/move/navigatin_48.gif" width="9"
|
||
height="8"/></td>
|
||
<td width="100%" bgcolor="#000000">
|
||
<table border="0" cellspacing="0" cellpadding="0">
|
||
<tr>
|
||
<td nowrap="nowrap" align="center">
|
||
<div align="center"
|
||
style="font-size:4px;padding:0;border:solid black 0; text-align:center"
|
||
id="prcont"></div>
|
||
<script language="javascript"
|
||
type="text/javascript">
|
||
let s = "";
|
||
for (i = 1; i <= 32; i++) {
|
||
s += '<span id="progress' + i + '"> </span>';
|
||
if (i < 32) {
|
||
s += ' '
|
||
}
|
||
}
|
||
document.getElementById('prcont').innerHTML = s;
|
||
</script>
|
||
</td>
|
||
</tr>
|
||
</table>
|
||
</td>
|
||
<td><img src="i/move/navigatin_50.gif" width="7"
|
||
height="8"/></td>
|
||
</tr>
|
||
</table>
|
||
</td>
|
||
</tr>
|
||
<tr>
|
||
<td>
|
||
<table width="100%" border="0" cellspacing="0" cellpadding="0">
|
||
<tr>
|
||
<td><img src="i/move/navigatin_51.gif" width="31"
|
||
height="8"/></td>
|
||
</tr>
|
||
<tr>
|
||
<td><img src="i/move/navigatin_54.gif" width="9"
|
||
height="20"/><img
|
||
src="i/move/navigatin_55i.gif" width="22"
|
||
height="20"
|
||
border="0"/></td>
|
||
</tr>
|
||
<tr>
|
||
<td>
|
||
<a onclick="return check('m7');" <? if ($rooms[$rhar[$user->getRoom()][4]]) {
|
||
echo 'id="m7"';
|
||
} ?> href="?rnd=0.817371946556865&path=4"><img
|
||
src="i/move/navigatin_59<? if (!$rooms[$rhar[$user->getRoom()][4]]) {
|
||
echo 'i';
|
||
} ?>.gif" width="21" height="20" border="0"
|
||
o<? if (!$rooms[$rhar[$user->getRoom()][4]]) {
|
||
echo 'i';
|
||
} ?>nmousemove="fastshow2('<?= $rooms[$rhar[$user->getRoom()][4]] ?>');"
|
||
onmouseout="hideshow();"/></a><img
|
||
src="i/move/navigatin_60.gif" width="10"
|
||
height="20"
|
||
border="0"/></td>
|
||
</tr>
|
||
<tr>
|
||
<td><img src="i/move/navigatin_63.gif" width="11"
|
||
height="21"/><img
|
||
src="i/move/navigatin_64i.gif" width="20"
|
||
height="21"
|
||
border="0"/></td>
|
||
</tr>
|
||
<tr>
|
||
<td><img src="i/move/navigatin_68.gif" width="31"
|
||
height="8"/></td>
|
||
</tr>
|
||
</table>
|
||
</td>
|
||
<td>
|
||
<table width="100%" border="0" cellspacing="0" cellpadding="0">
|
||
<tr>
|
||
<td>
|
||
<a onclick="return check('m1');" <? if ($rooms[$rhar[$user->getRoom()][1]]) {
|
||
echo 'id="m1"';
|
||
} ?> href="?rnd=0.817371946556865&path=1"><img
|
||
src="i/move/navigatin_52<? if (!$rooms[$rhar[$user->getRoom()][1]]) {
|
||
echo 'i';
|
||
} ?>.gif" width="19" height="22" border="0"
|
||
<? if (!$rooms[$rhar[$user->getRoom()][1]]) {
|
||
echo 'i';
|
||
} ?>onmousemove="fastshow2('<?= $rooms[$rhar[$user->getRoom()][1]] ?>');"
|
||
onmouseout="hideshow();"/></a></td>
|
||
</tr>
|
||
<tr>
|
||
<td><a href="?rnd=0.817371946556865"><img
|
||
src="i/move/navigatin_58.gif"
|
||
width="19" height="33"
|
||
border="0" o
|
||
nmousemove="fastshow2('<strong>Обновить</strong><br />Переходы:<br />Картинная галерея 1<br />Зал ораторов<br />Картинная галерея 3');"
|
||
onmouseout="hideshow();"/></a>
|
||
</td>
|
||
</tr>
|
||
<tr>
|
||
<td>
|
||
<a onclick="return check('m5');" <? if ($rooms[$rhar[$user->getRoom()][3]]) {
|
||
echo 'id="m5"';
|
||
} ?> href="?rnd=0.817371946556865&path=3"><img
|
||
src="i/move/navigatin_67<? if (!$rooms[$rhar[$user->getRoom()][3]]) {
|
||
echo 'i';
|
||
} ?>.gif" width="19" height="22" border="0"
|
||
<? if (!$rooms[$rhar[$user->getRoom()][3]]) {
|
||
echo 'i';
|
||
} ?>onmousemove="fastshow2('<?= $rooms[$rhar[$user->getRoom()][3]] ?>');"
|
||
onmouseout="hideshow();"/></a></td>
|
||
</tr>
|
||
</table>
|
||
</td>
|
||
<td>
|
||
<table width="100%" border="0" cellspacing="0" cellpadding="0">
|
||
<tr>
|
||
<td><img src="i/move/navigatin_53.gif" width="30"
|
||
height="8"/></td>
|
||
</tr>
|
||
<tr>
|
||
<td><img src="i/move/navigatin_56i.gif" width="21"
|
||
height="20"
|
||
border="0"/><img src="i/move/navigatin_57.gif"
|
||
width="9"
|
||
height="20"/></td>
|
||
</tr>
|
||
<tr>
|
||
<td><img src="i/move/navigatin_61.gif" width="8"
|
||
height="21"/><a
|
||
onclick="return check('m3');" <? if ($rooms[$rhar[$user->getRoom()][2]]) {
|
||
echo 'id="m3"';
|
||
} ?> href="?rnd=0.817371946556865&path=2"><img
|
||
src="i/move/navigatin_62<? if (!$rooms[$rhar[$user->getRoom()][2]]) {
|
||
echo 'i';
|
||
} ?>.gif" width="22" height="21" border="0"
|
||
<? if (!$rooms[$rhar[$user->getRoom()][2]]) {
|
||
echo 'i';
|
||
} ?>onmousemove="fastshow2('<?= $rooms[$rhar[$user->getRoom()][2]] ?>');"
|
||
onmouseout="hideshow();"/></a></td>
|
||
</tr>
|
||
<tr>
|
||
<td><img src="i/move/navigatin_65i.gif" width="21"
|
||
height="20"
|
||
border="0"/><img src="i/move/navigatin_66.gif"
|
||
width="9"
|
||
height="20"/></td>
|
||
</tr>
|
||
<tr>
|
||
<td><img src="i/move/navigatin_69.gif" width="30"
|
||
height="8"/></td>
|
||
</tr>
|
||
</table>
|
||
</td>
|
||
</tr>
|
||
</table>
|
||
</td>
|
||
</tr>
|
||
</table>
|
||
</td>
|
||
</tr>
|
||
</table>
|
||
<div id="mmoves"
|
||
style="background-color:#FFFFCC; visibility:hidden; overflow:visible; position:absolute; border-color:#666666; border-style:solid; border-width: 1px; padding: 2px; white-space: nowrap;"></div>
|
||
<script language="javascript" type="text/javascript">
|
||
const progressEnd = 32;
|
||
let progressColor = '#00CC00';
|
||
let mtime = parseInt('<?=($_SESSION["time"] - time())?>');
|
||
if (!mtime || mtime <= 0) {
|
||
mtime = 0;
|
||
}
|
||
const progressInterval = Math.round(mtime * 1000 / progressEnd);
|
||
let is_accessible = true;
|
||
let progressAt = progressEnd;
|
||
let progressTimer;
|
||
|
||
function progress_clear() {
|
||
for (let i = 1; i <= progressEnd; i++) {
|
||
document.getElementById('progress' + i).style.backgroundColor = 'transparent';
|
||
}
|
||
progressAt = 0;
|
||
for (let t = 1; t <= 8; t++) {
|
||
if (document.getElementById('m' + t)) {
|
||
const tempname = document.getElementById('m' + t).children[0].src;
|
||
if (tempname.match(/b\.gif$/)) {
|
||
document.getElementById('m' + t).children[0].id = 'backend';
|
||
}
|
||
let newname;
|
||
newname = tempname.replace(/(b)?\.gif$/, 'i . gif');
|
||
document.getElementById('m' + t).children[0].src = newname;
|
||
}
|
||
}
|
||
is_accessible = false;
|
||
set_moveto(true);
|
||
}
|
||
|
||
function progress_update() {
|
||
progressAt++;
|
||
if (progressAt > progressEnd) {
|
||
for (let t = 1; t <= 8; t++) {
|
||
if (document.getElementById('m' + t)) {
|
||
let tempname = document.getElementById('m' + t).children[0].src;
|
||
let newname;
|
||
newname = tempname.replace(/i\.gif$/, ' . gif');
|
||
if (document.getElementById('m' + t).children[0].id == 'backend') {
|
||
tempname = newname.replace(/\.gif$/, 'b . gif');
|
||
newname = tempname;
|
||
}
|
||
document.getElementById('m' + t).children[0].src = newname;
|
||
}
|
||
}
|
||
is_accessible = true;
|
||
if (window.solo_store && solo_store) {
|
||
solo(solo_store);
|
||
}
|
||
set_moveto(false);
|
||
} else {
|
||
document.getElementById('progress' + progressAt).style.backgroundColor = progressColor;
|
||
progressTimer = setTimeout('progress_update()', progressInterval);
|
||
}
|
||
}
|
||
|
||
function set_moveto(val) {
|
||
document.getElementById('moveto').disabled = val;
|
||
if (document.getElementById('bmoveto')) {
|
||
document.getElementById('bmoveto').disabled = val;
|
||
}
|
||
}
|
||
|
||
function progress_stop() {
|
||
clearTimeout(progressTimer);
|
||
progress_clear();
|
||
}
|
||
|
||
function check(it) {
|
||
return is_accessible;
|
||
}
|
||
|
||
function check_access() {
|
||
return is_accessible;
|
||
}
|
||
|
||
function ch_counter_color(color) {
|
||
progressColor = color;
|
||
for (let i = 1; i <= progressAt; i++) {
|
||
document.getElementById('progress' + i).style.backgroundColor = progressColor;
|
||
}
|
||
}
|
||
|
||
if (mtime > 0) {
|
||
progress_clear();
|
||
progress_update();
|
||
} else {
|
||
for (var i = 1; i <= progressEnd; i++) {
|
||
document.getElementById('progress' + i).style.backgroundColor = progressColor;
|
||
}
|
||
}
|
||
</script>
|
||
</TD>
|
||
</TR>
|
||
</TABLE>
|
||
<br/>
|
||
Всего живых участников на данный момент : <b><? echo "<b>" . $kol_pl . "</b> + <b>" . $ls . "</b>"; ?></b>...<br/>
|
||
<div id="hint3" class="ahint"></div>
|
||
<script>top.onlineReload(true)</script>
|