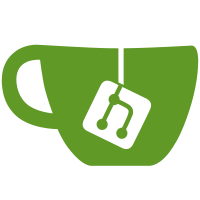
Singleton в некоторых местах вместо решения #42. Новые шаги для решения #16 и #52. Closes #42. Closes #32. Closes #31.
45 lines
1.6 KiB
PHP
45 lines
1.6 KiB
PHP
<?php
|
||
|
||
|
||
namespace Battles\Magic;
|
||
|
||
use Battles\Database\Db;
|
||
use Battles\DressedItems;
|
||
use Battles\Item;
|
||
use Battles\User;
|
||
|
||
class Sharpen extends Magic
|
||
{
|
||
private int $magicDifficulty;
|
||
|
||
/**
|
||
* Sharpen constructor.
|
||
*
|
||
* @param int $sharpenStrength
|
||
* @param int $magicDifficulty
|
||
*
|
||
*/
|
||
public function __construct(int $sharpenStrength, int $magicDifficulty)
|
||
{
|
||
$this->magicDifficulty = $magicDifficulty;
|
||
if (!$this->isUsable()) {
|
||
return $this->status;
|
||
}
|
||
$item = DressedItems::getDressedItemBySlot(Item::ITEM_TYPE_WEAPON, $_SESSION['uid']);
|
||
// Проверяем, что в названии предмета нет цифр и плюсов.
|
||
if (preg_match('/[\W\S]+\+\[?[\d]]?/', $item->name)) {
|
||
return 'Этот предмет точить нельзя!';
|
||
}
|
||
$newMinPhysicalDamage = $item->add_min_physical_damage + $sharpenStrength;
|
||
$newMaxPhysicalDamage = $item->add_max_physical_damage + $sharpenStrength;
|
||
$newItemName = $item->name . " [+$sharpenStrength]";
|
||
|
||
Db::getInstance()->execute('UPDATE battles.inventory SET name = ?, add_min_physical_damage = ?, add_max_physical_damage = ? WHERE item_id = ? ', [$newItemName, $newMinPhysicalDamage, $newMaxPhysicalDamage, $item->item_id]);
|
||
return "У вас получилось изготовить предмет $newItemName!";
|
||
}
|
||
|
||
private function isUsable(): bool
|
||
{
|
||
return $this->isNotInBattle(User::getInstance()) && $this->isSuccess(User::getInstance(), $this->magicDifficulty);
|
||
}
|
||
} |