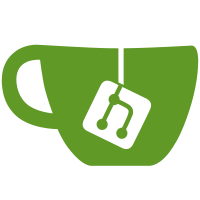
Singleton в некоторых местах вместо решения #42. Новые шаги для решения #16 и #52. Closes #42. Closes #32. Closes #31.
48 lines
1.8 KiB
PHP
48 lines
1.8 KiB
PHP
<?php
|
|
// Магия восстановления здоровья
|
|
use Battles\Magic\Magic;
|
|
use Battles\User;
|
|
use Battles\Database\Db;
|
|
|
|
class Healing extends Magic
|
|
{
|
|
private $target;
|
|
private $magicPower;
|
|
|
|
/**
|
|
* Магия лечения.
|
|
* @param $target - кого лечим.
|
|
* @param $power - на сколько лечим.
|
|
* @param null $isPercentage - если включён, считает $power в процентах, иначе, по-умолчанию просто как число.
|
|
*/
|
|
public function __construct($target, $power, $isPercentage = null)
|
|
{
|
|
$this->magicPower = $power;
|
|
$this->target = $target;
|
|
if (!$this->isUsable()) {
|
|
return $this->status;
|
|
}
|
|
if ($isPercentage) {
|
|
$healHealthAmount = $this->target->health + $this->target->maxHealth / 100 * $this->magicPower;
|
|
} else {
|
|
$healHealthAmount = $this->target->health + $this->magicPower;
|
|
}
|
|
if ($healHealthAmount > $this->target->maxHealth) {
|
|
$healHealthAmount = $this->target->maxHealth;
|
|
}
|
|
Db::getInstance()->execute('UPDATE users SET health = ? WHERE id = ?', [$healHealthAmount, $this->target->id]);
|
|
$targetName = $this->target->login;
|
|
return "Вы восстановили ${healHealthAmount} здоровья персонажу ${targetName}.";
|
|
}
|
|
|
|
/**
|
|
* Проверки на успех.
|
|
* @return bool
|
|
*/
|
|
private function isUsable(): bool
|
|
{
|
|
$this->target = $this->target == $_SESSION['uid'] ? User::getInstance() : User::getInstance($this->target);
|
|
return $this->isVisible(User::getInstance(), $this->target) && $this->isNotDead(User::getInstance()) && $this->enoughMana(User::getInstance()) && $this->isSuccess(User::getInstance());
|
|
}
|
|
}
|