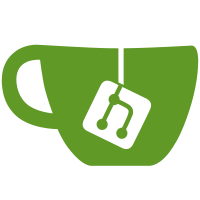
# Conflicts: # admin/admin.php # classes/Battles/User.php # classes/Battles/UserStats.php # fbattle.php # functions.php
90 lines
2.7 KiB
PHP
90 lines
2.7 KiB
PHP
<?php
|
||
/**
|
||
* Author: lopiu
|
||
* Date: 06.07.2020
|
||
* Time: 22:41
|
||
*/
|
||
|
||
namespace Battles;
|
||
|
||
use Battles\Database\Db;
|
||
use Battles\Models\Inventory;
|
||
use stdClass;
|
||
|
||
class DressedItems
|
||
{
|
||
private int $USERID;
|
||
private stdClass $dressedItem;
|
||
private static Db $db;
|
||
private object $dressed;
|
||
|
||
/**
|
||
* DressedItems constructor.
|
||
* @param int $user_id ID игрока.
|
||
*/
|
||
public function __construct(int $user_id)
|
||
{
|
||
self::$db = Db::getInstance();
|
||
$this->USERID = $user_id;
|
||
$this->dressed = self::$db->ofetchAll('select * from inventory where dressed_slot > 0 and owner_id = ?', $this->USERID);
|
||
}
|
||
|
||
public static function getDressedItemBySlot($itemSlot, $ownerId)
|
||
{
|
||
return self::$db->ofetch('SELECT *, COUNT(1) AS count FROM inventory WHERE owner_id = ? AND dressed_slot = ?', [$ownerId, $itemSlot]);
|
||
}
|
||
|
||
public function getItemsInSlots(): stdClass
|
||
{
|
||
$this->dressedItem = new stdClass();
|
||
foreach ($this->dressed as $item) {
|
||
$i = $item->dressed_slot;
|
||
$this->dressedItem->$i = $item;
|
||
}
|
||
return $this->dressedItem;
|
||
}
|
||
|
||
/**
|
||
* Снимает с предмета статус одетого на персонажа в определённом слоте персонажа.
|
||
* @param $slot_id - номер слота.
|
||
*/
|
||
public function undressItem($slot_id)
|
||
{
|
||
self::getItemsInSlots();
|
||
// Проверяем, что используется один из 12 слотов и наличие предмета в слоте.
|
||
if (in_array($slot_id, Item::ITEM_TYPES_ALLOWED_IN_SLOTS) && $this->dressedItem->$slot_id) {
|
||
Inventory::undressOne($slot_id, $this->USERID);
|
||
}
|
||
}
|
||
|
||
public static function undressAllItems($user_id)
|
||
{
|
||
return self::$db->execute('UPDATE inventory SET dressed_slot = 0 WHERE dressed_slot BETWEEN 1 AND 12 AND owner_id = ?', $user_id);
|
||
}
|
||
|
||
public function checkRequirements()
|
||
{
|
||
$stats = (new UserStats($this->USERID))->getFullStats();
|
||
$q = 'select count(*) from inventory where
|
||
dressed_slot > 0 and
|
||
need_strength > ? and
|
||
need_dexterity > ? and
|
||
need_intuition > ? and
|
||
need_endurance > ? and
|
||
need_intelligence > ? and
|
||
need_wisdom > ? and
|
||
owner_id = ?';
|
||
$args = [
|
||
$stats->strength,
|
||
$stats->dexterity,
|
||
$stats->intuition,
|
||
$stats->endurance,
|
||
$stats->intelligence,
|
||
$stats->wisdom,
|
||
$this->USERID
|
||
];
|
||
if (self::$db->fetchColumn($q, $args) > 0) {
|
||
self::undressAllItems($this->USERID);
|
||
}
|
||
}
|
||
} |